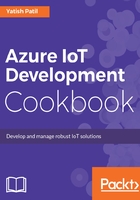
上QQ阅读APP看书,第一时间看更新
How to do it...
In this section, we will start with registering the new device, followed by some more device management operations such as: retrieve, delete, list, export, and import.
- Create a device identity by initializing the Azure IoT Hub registry connection:
string deviceId = "myFirstDevice";
registryManager = RegistryManager.CreateFromConnectionString(connectionString);
Device device = new Device();
try
{
device = await registryManager.AddDeviceAsync(new Device(deviceId));
success = true;
}
catch (DeviceAlreadyExistsException)
{
success = false;
}
- Retrieve the device identity by deviceId:
string deviceId = "myFirstDevice";
Device device = new Device();
try
{
device = await registryManager.GetDeviceAsync(deviceId);
}
catch (DeviceAlreadyExistsException)
{
return device;
}
- Delete the device identity:
string deviceId = "myFirstDevice";
Device device = new Device();
try
{
device = GetDevice(deviceId);
await registryManager.RemoveDeviceAsync(device);
success = true;
}
catch (Exception ex)
{
success = false;
}
- Disable the IoT device from the device registry. This can be handled through an IoT solution in certain scenarios, such as if the device is tampered with or these are some critical issues with the device:
string deviceId = "myFirstDevice";
Device device = new Device();
try
{
device = GetDevice(deviceId);
device.Status = DeviceStatus.Disabled;
// Update the device registry
await registryManager.UpdateDeviceAsync(device);
success = true;
}
catch (Exception ex)
{
success = false;
}
- List up to 1000 identities:
try
{
var devicelist = registryManager.GetDevicesAsync(1000);
return devicelist.Result;
}
catch (Exception ex)
{
//
- Export all the identities to Azure Blob storage:
var blobClient = storageAccount.CreateCloudBlobClient();
string Containername = "iothubdevices";
//Get a reference to a container
var container = blobClient.GetContainerReference(Containername);
container.CreateIfNotExists();
//Generate a SAS token
var storageUri = GetContainerSasUri(container);
await registryManager.ExportDevicesAsync(storageUri, "devices1.txt", false);
}
- Import all the identities to Azure Blob storage:
await registryManager.ImportDevicesAsync(storageUri, OutputStorageUri);
The output of ImportDevice methods creates a job which can be used to perform bulk operation like create and delete.