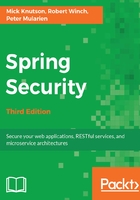
Expression-based authorization
You may have noticed that granting access to everyone was not nearly as concise as we may have liked. Fortunately, Spring Security can leverage Spring Expression Language (SpEL) to determine whether a user has authorization. In the following code snippet, you can see the updates when using SpEL with Spring Security:
//src/main/java/com/packtpub/springsecurity/configuration/
SecurityConfig.java
http.authorizeRequests()
.antMatchers("/").access("hasAnyRole('ANONYMOUS', 'USER')")
.antMatchers("/login/*").access("hasAnyRole('ANONYMOUS', 'USER')")
.antMatchers("/logout/*").access("hasAnyRole('ANONYMOUS', 'USER')")
.antMatchers("/admin/*").access("hasRole('ADMIN')")
.antMatchers("/events/").access("hasRole('ADMIN')")
.antMatchers("/**").access("hasRole('USER')")
You may notice that the /events/ security constraint is brittle. For example, the /events URL is not protected by Spring Security to restrict the ADMIN role. This demonstrates the need to ensure that we provide multiple layers of security. We will exploit this sort of weakness in Chapter 11, Fine-Grained Access Control.
Changing the access attribute from hasAnyRole('ANONYMOUS', 'USER') to permitAll() might not seem like much, but this only scratches the surface of the power of Spring Security's expressions. We will go into much greater detail about access control and Spring expressions in the second half of the book. Go ahead and verify that the updates work by running the application.
Your code should now look like chapter02.04-calendar.