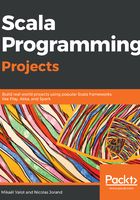
Class inheritance
Scala classes are extensible. You can extend an existing class to inherit from all its members. If B extends A, we say that B is a subclass of A, a derivation of B, or a specialization of B. A is a superclass of B or a generalization of B.
Let's see how it works in an example. Type the following code in the worksheet:
class Shape(val x: Int, val y: Int) {
val isAtOrigin: Boolean = x == 0 && y == 0
}
class Rectangle(x: Int, y: Int, val width: Int, val height: Int)
extends Shape(x, y)
class Square(x: Int, y: Int, width: Int)
extends Rectangle(x, y, width, width)
class Circle(x: Int, y: Int, val radius: Int)
extends Shape(x, y)
val rect = new Rectangle(x = 0, y = 3, width = 3, height = 2)
rect.x
rect.y
rect.isAtOrigin
rect.width
rect.height
The classes Rectangle and Circle are subclasses of Shape. They inherit from all the members of Shape: x, y, and isAtOrigin. This means that when I instantiate a new Rectangle, I can call members declared in Rectangle, such as width and height, and I can also call members declared in Shape.
When declaring a subclass, you need to pass the constructor arguments of the superclass, as if you were instantiating it. As Shape declares two constructor parameters, x and y, we have to pass them in the declaration extends Shape(x, y). In this declaration, x and y are themselves the constructor arguments of Rectangle. We just passed these arguments up the chain.
Notice that in the subclasses, the constructor parameters x and y are declared without val. If we had declared them with val, they would have been promoted as publicly available attributes. The problem is that Shape also has x and y as public attributes. In this situation, the compiler would have raised a compilation error to highlight the conflict.