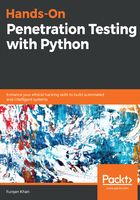
Classes and objects
A class can be thought of as a template or a blueprint that contains the definition of the method and the variables that are to be used with objects of that class. An object is nothing but an instance of the class, which contains actual values rather than variables. A class can also be defined as a collection of objects.
To put this in simple terms, a class is a collection of variables and methods. The methods actually define the behavior or the operations that the class performs and the variables are the entities upon which the operations are performed. In Python, a class is declared with the class keyword, followed by the class name. The following example shows how to declare a basic employee class, along with some methods and operations. Let's create a Python script called Classes.py:

The following bullet points explain the preceding code and its structure:
- class Id_Generator(): In order to declare a class in Python, we need to associate it with the class keyword, which is what we did in line 2 of the code. Whatever proceeds at an equal indentation forms part of the Id_Generator class. The purpose of this class is to generate an employee ID for every new employee created. It does this with the generate() method.
- def __init__(self): Every class in Python or any other programming language has got a constructor. This is either explicitly declared or it is not declared and the default constructor is taken implicitly. If you come from a background of using Java or C++, you might be used to the name of the constructor being the same as the class name, but this is not always the case. In Python, the class constructor method is defined using the __init__ word, and it always takes self as an argument.
- self: The self is similar to a keyword. The self in Python represents the current instance of the class, and in Python every class method which is an instance method must have self as its first argument. This also applies to the constructor. It should be noted that while invoking the instance method, we don't need to explicitly pass the instance of a class as an argument; Python implicitly takes care of this for us. Any instance-level variable has to be declared with the self keyword. This can be seen in the constructor—we have declared an instance variable ID as self.id and initialized it to 0.
- def generate(self): The generate is an instance method that increments the ID and returns the incremented ID.
- class Employee(): The employee class is a class that is used to create employees with its constructor. It prints the details of the employees with the printDetails method.
- def __init__(self,Name,id_gen): There can be two kinds of constructor – parameterized and unparameterized. Any constructor that takes parameters is a parameterized constructor. Here, the constructor of the employee class is parameterized, because it takes two parameters: the name of the employee to be created and the instance of the Id_Generator class. In this method, we just call the generate method of the Id_Generator class, which returns us the employee ID. The constructor also initializes the employee name that was passed to the self class instance variable, which is name. It also initializes the other variables, D_id and Salary, to None.
- def printDetails(self): This is the method that will print the details of the employee.
- Lines 24–32: In this section of the code, we first create the instance of the Id_Generator class and name it Id_gen. Then, we create an instance of the Employee class. Remember that the constructor of the class is invoked at the moment in which we create the instance of the class. Since in this case the constructor is parameterized, we have to create an instance that takes two parameters, with the first being the employee name and the second being the instance of the Id_Generator class. This is what we did in line 25: emp1=Employee('Emp1',Id_gen). As mentioned earlier, we don't need to pass self explicitly; Python takes care of this implicitly. After that, we assign some values to the Salary and D_id instance variables of the employee class for the Emp1 instance. We also create another employee called Emp2, as shown in line 28. Finally, we print the details of both the employees by invoking emp1.printDetails() and emp2.printDetails().