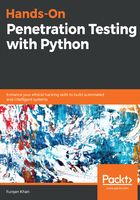
A closer look at for loops
The for loops in Python exceed the capabilities of for loops in other programming languages. When calling upon iterables such as strings, tuples, lists, sets, or dictionaries, the for loop internally calls the iter to get an iterator. Then, it calls the next method over that iterator to get the actual element in the iterable. It then calls next repeatedly until a StopIteration exception is raised, which it would internally handle and get us out of the loop. The syntax of the for loop is given as follows:
for var in iterable:
statement 1
statement 2
statement n
Let's create a file called for_loops.py, which will explain the basic use of for loops:

In the preceding example, we used the Python range function/method, which helps us implement the traditional for loop that we learnt in other programming languages such as C, C ++, or Java. This might look like for i =0 ;i < 5 ;i ++. The range function in Python takes one mandatory argument and two default ones. The mandatory argument specifies the limit of the iteration and, starting from index 0, returns numbers until it reaches the limit, as seen in lines 3 and 4 of the code. When invoked with two arguments, the first one serves as the starting point of the range and the last one serves as the end point, as is depicted in lines 7 and 8 of our code. Finally, when the range function is invoked with three arguments, the third one serves as the step size, which is equal to one by default. This is depicted in the following output and lines 12 and 13 of the sample code:

Let's take a look at another example of a for loop, which we shall use to iterate over all the iterables that Python defines. This will allow us to explore the real power of for loops. Let's create a file called for_loops_ad.py:

Earlier, we saw how we can read the values from lists, strings, and tuples. In the preceding example, we use the for loop to enumerate over strings, lists, and dictionaries. We learned earlier that the for loop actually invokes the iter method of iterables and then calls the next method for each iteration. This is shown in the following example:
